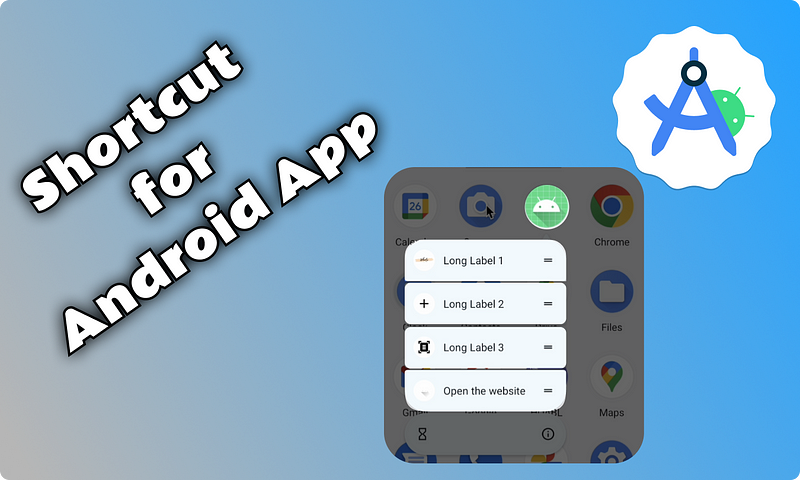
Hello Buddy’s
Let’s start with by adding dependencies.
Add the below dependency to your app/build.gradle.kts file.
implementation ("androidx.core:core:1.13.1")
implementation ("androidx.core:core-google-shortcuts:1.1.0")
Then go to your app/src/main/AndroidManifest.xml file and add the below meta tag inside your <activity></activity> tag.
<meta-data android:name="android.app.shortcuts"
android:resource="@xml/shortcuts"/>
After this you need to create a @xml/shortcuts file inside your res/xml folder. In the below xml file we are going to define a static shortcut for your application. if you want to create a static permenent shortcut you can add the shortcut in this xml file.
And you have to add the action Intent also for navigation to the particular activity. Make sure the targetPackage name is your app package name
android:targetPackage="com.task.master"
then only the android os will find your application. And make sure your have given the unique Id for shortcuts.
<shortcuts xmlns:android="http://schemas.android.com/apk/res/android">
<shortcut
android:shortcutId="shortcut_1"
android:enabled="true"
android:icon="@drawable/tm_logo"
android:shortcutShortLabel="@string/ShortCutShortLabel_1"
android:shortcutLongLabel="@string/ShortCutLongLabel_1"
android:shortcutDisabledMessage="@string/ShortCutDisableLabel_1"
>
<intent
android:action="android.intent.action.VIEW"
android:targetPackage="com.task.master"
android:targetClass="com.task.master.presentation.ui.activities.MainActivity"
>
<extra
android:name="Type"
android:value="Create"
/>
</intent>
<categories android:name="android.shortcut.conversation"/>
<capability-binding android:key="action.intent.CREATE_MESSAGE"/>
</shortcut>
<shortcut
android:shortcutId="shortcut_2"
android:enabled="true"
android:icon="@drawable/tm_logo"
android:shortcutShortLabel="@string/ShortCutShortLabel_2"
android:shortcutLongLabel="@string/ShortCutLongLabel_2"
android:shortcutDisabledMessage="@string/ShortCutDisableLabel_2"
>
<intent
android:action="android.intent.action.VIEW"
android:targetPackage="com.task.master"
android:targetClass="com.task.master.presentation.ui.activities.MainActivity"
>
<extra
android:name="Type"
android:value="View"
/>
</intent>
<categories android:name="android.shortcut.conversation"/>
<capability-binding android:key="action.intent.CREATE_MESSAGE"/>
</shortcut>
</shortcuts>
Once you added the files and code to your android application your app will show the shortcut which you defined in the xml/shortcuts.xml.
Now I’m going to show you how to add the shortcuts dynamically from the activity. So, you can add shortcut dynamically using ShortcutInfoCompat and push that by using ShortcutManagerCompat check the below code to add the dynamic shortcuts.
val context = this.baseContext
val shortcut = ShortcutInfoCompat.Builder(context, "dynamic_Id")
.setShortLabel("Dynamic Item")
.setLongLabel("Dynamic Item")
.setIcon(IconCompat.createWithResource(context, R.drawable.profile_icon))
.setIntent(
Intent(context,MainActivity::class.java).apply {
action = Intent.ACTION_VIEW
putExtra("Type", "")
}
)
.build()
ShortcutManagerCompat.pushDynamicShortcut(context, shortcut)
After this step you can add the shortcuts from any activity and based on the server response also you can add the shortcut in your application.
Conclusion
By using shortcut, we can create better user interaction and faster navigation to the particular section for the users.
You can find the source code here
Thank you
Comments
Post a Comment